In the past, everytime I created a script I made the same changes – I removed the comments around Start and Update and added some regions to organize my code. Standard housekeeping stuff, or put another way, tedious and repetitive work, exactly the type of work every dev dislikes. I wanted a better way.
When I Googled the problem I found a myriad of solutions, some simple (copy and paste) and some so convoluted it wasn’t worth the effort (who needs an Editor plug-in and custom Editor scripts just to make custom templates?!). The most straightforward solution involved “simply” updating the Unity default template and adding new templates to the same folder. Doing it that way created a brittle solution that would need constant tinkering everytime I upgraded Unity. Ugg, no thanks.
Then fortune shined on me and I found this tweet by @UnityBerserkers. I took a good look at the picture, and discovered they had a ScriptTemplates folder under the Assets folder and said it worked. Guess what, they were right. I replicated their solution using Unity 2019.3.1f1. As an added benefit, you can commit the customizations to your version control so the whole team always uses the same templates.
You can read all the details or just jump right to the step-by-step process.
Custom ScriptTemplates For Each Project
Create a ScriptTemplates Folder
Since the @UnityBerserkers tweet only gave a high-level overview of the solution, I followed up with more research and did some trial-and-error of my own. I found what worked and more importantly, how it worked. I didn’t want to just copy a solution, I wanted to understand it so I could customize it better.
First off, you need to create a ScriptTemplates
folder as a direct child of the Assets
folder. I always create a _Game
folder for my game assets to separate them from downloaded/imported assets. Initially, I tried creating the new folder in my _Game
folder, however, it didn’t work.

Some Strict Naming Conventions
In your new ScriptTemplates
folder you can create any number of script templates, the gotcha – they must follow a strict naming convention that, in true Unity fashion, is not well documented. I’ll use the following file names as examples and break down what each part means.
- 80-WBD C# Script__MB Script-NewBehaviourScript.cs.txt
- 80-WBD C# Script__UCO Script-NewCsharpScript.cs.txt

Each file starts with a number, I used 80 in my example. it might appear random, however, using a custom sorting number is fairly common when you don’t want to sort alphabetically. Of course, the sorting numbers are not well documented, you can read Red Blue Games‘ section on Sort Order for Built-in Items for more details. For simplicity, the default C# Script
uses 81, so you can use 80 to put your templates before Unity’s, 82 to put them after. I believe you can hide Unity’s templates by using 81 but I haven’t tested that.
After the sorting number you need a single dash “-“, and there should be no spaces between the dash and the Primary Menu Name.
I currently have two templates, so I used a menu/sub-menu structure. The primary menu name comes immediately after the dash. In my example, I used “WBD C# Script”. After that comes two underscore characters “__” – make sure you use 2 of them. After the underscores you can enter another folder name or the menu item name. I choose the menu item name of “MB Script” and “UCO Script”.

After that, add another single dash, and again no spaces before the next section.
Now comes the default filename when Unity creates the code file. To me, this is the least important part of the name. You immediately rename the file once Unity creates it.
Lastly, you add the extension and in this case a double extension. The template itself needs to be a text file (.txt), however, Unity uses it to create a C# file (.cs) so you have to include both. Note, that Unity does not display the “.txt” extension in the Editor, however, you can see it in the explorer (assuming you have file extensions turned on).


Template Creation
Now comes the easy part, open the text file and create your script template. Check out this gist for an example. In practice, you only have to worry about two text replacements, however, a third one exists so I will briefly explained it below.
The first text replacement, #SCRIPTNAME#
, not surprisingly takes the filename and makes it the object/class name. It is important the filename matches the name, and this ensures that happens upon creation.
The second text replacement, #NOTRIM#
, prevents editors from auto-stripping blank spaces by accident. You use this to create correctly indented but empty lines as placeholders. The lines in Start and Update are good examples.
The third text replacement, #SCRIPTNAME_LOWER#
, creates a lowercase version of the script name. I don’t use it, but if you do know that you will get either an all lowercase version of the filename or one prefixed with “my”.
Here is a copy of my default “MB Script”.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
namespace SpaceMonkeys
{
public class #SCRIPTNAME# : MonoBehaviour
{
#region Members
#endregion Members
#region MonoBehaviours
void Start()
{
#NOTRIM#
}
void Update()
{
#NOTRIM#
}
#endregion MonoBehaviours
#region Properties
#endregion Properties
#region Methods
#endregion Methods
#region Helpers
#endregion Helpers
}
}
Restart Unity
Unity creates its menus on start up, so any changes require you restarting Unity.
Optional – Commit to Version Control
If you use version control then as a bonus these get committed as part of the project. That means everyone on your team will use the same templates. If you had added/changed the templates in the Unity folder this would not be possible.
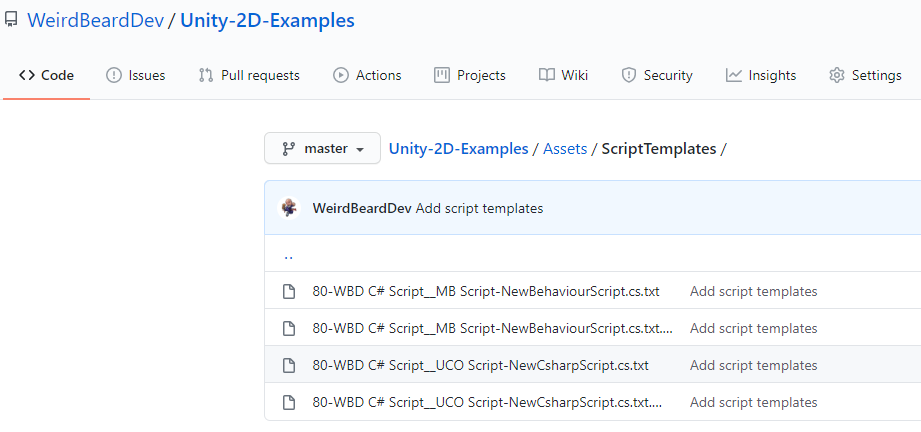
Step-by-Step Process
NOTE: I tested this solution with Unity 2019.3.1f1.
- Create a
ScriptTemplates
folder as a direct child of theAssets
folder - Create an empty text file that follows a specific naming convention
- Start with 80 if you want it before C# Script and 82 if you want it after, example: “80”
- Add a single dash, example: “80-“
- Add a menu name, example: “80-WBD C# Script”
- Add two underscores, example: “80-WBD C# Script__”
- Add a sub-menu name, example: “80-WBD C# Script__MB Script”
- Add a single dash, example: “80-WBD C# Script__MB Script-“
- Add a default file name, example: “80-WBD C# Script__MB Script-NewBehaviourScript”
- Add the double file extensions, example: “80-WBD C# Script__MB Script-NewBehaviourScript.cs.txt”
- Open the text file and create your default script template
- Use
#SCRIPTNAME#
after the object type, e.g., class, enum, etc. - Use
#NOTRIM#
to create empty lines with proper indentation - Type up your remaining template as desired
- See this gist for an example
- Use
- Restart Unity
- Optional: commit to your version control system so your team uses the same templates
If you try this in another version of Unity please leave me a comment and let me know.
Life’s an adventure, enjoy your quest!
Send a Missive